Since I often download pdfs, sourcecode and other files from the web, I often end up with my downloads folder being cluttered with full and empty folders between each other.
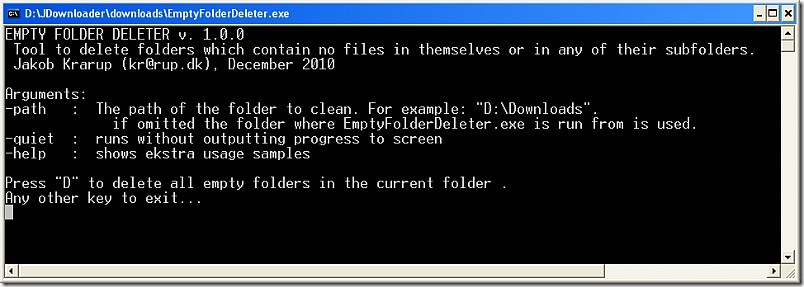
For that reason I made a little commandline tool to delete empty folders. I have it in my downloads folder, so I can doubleclick it whenever I’ve been sorting stuff, and want the empty folders deleted. To be sure that you meant to run the program it asks you to press D(elete) on the keyboard to continue. You can run it from a batch file with the parameter "-quiet" to make it run without confirmation.
You can download the application
(zip), or the source code
.
An interesting code snippet is the Options class. Earlier I've been using Plossum, but I didn't want to include referenced DLLs in my app, so I made the Options class as an alternative. It is a class which parses the command line and stores the options in public properties, so the logic in the command line version could easily be changed to accept input from for example an xml file. The main logic is in the constructor which receives the string[] sent from the Main() method of the program.cs.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
using System.Reflection;
namespace EmptyFolderDeleter
{
// ****************************************************************
// Jakob Krarup - December 2010
// http://www.xnafan.net
// Distributed under Creative Commons Attribution license:
// http://creativecommons.org/licenses/by/3.0/
// Short version: use it how you wish, just leave my name on it 
// ****************************************************************
///
/// Class to parse commandline options for the EmptyFolderDeleter program
///
public class Options
{
#region Properties
///
/// The folder to delete files in
///
public string Folder { get; set; }
///
/// Whether to refrain from writing status to the console
///
public bool Quiet { get; set; }
///
/// Whether to display the program help for the user
///
public bool Help { get; set; }
///
/// Whether there was an error parsing the arguments in the constructor
///
public bool ParseError { get; set; }
///
/// What the error was, when parsing the arguments in the constructor
///
public string ParseErrorMessage { get; set; }
#endregion
///
/// Parses the commandline arguments and saves them in an Options object
///
///
A string[] containing the arguments
/// An Options object containing the parsed values
public Options(string[] args)
{
if (args.Length == 0)
{
return;
}
else
{
//convert the argument array to list to be able to use LINQ and to remove items
List argList = args.ToList();
//create a stringbuilder for the errormessages
StringBuilder error = new StringBuilder();
try
{
//if "help" is one of the arguments, set the help property of options to true
if (argList.Any(arg => arg.ToLower() == "-help"))
{
this.Help = true;
argList.Remove("-help");
}
//if "help" is one of the arguments, set the help property of options to true
if (argList.Any(arg => arg.ToLower() == "/?"))
{
this.Help = true;
argList.Remove("/?");
}
//if "quiet" is one of the arguments, set the verbose property to false
this.Quiet = argList.Any(arg => arg.ToLower() == "-quiet");
argList.Remove("-quiet");
if (argList.Any(arg => arg.StartsWith("-path")))
{
//find the first argument that begins with "-path"
string folderArgument = argList.First(arg => arg.StartsWith("-path"));
string folderPath = folderArgument.Split('=')[1].Trim();
//remove quotationmarks
this.Folder = folderPath.Replace("\"", "");
if (!Directory.Exists(this.Folder))
{
this.ParseError = true;
error.Append("The path '" + this.Folder + "' is not a valid folder.\r\n");
}
argList.Remove(folderArgument);
}
//if there are still arguments left, the user has entered an invalid argument
if (argList.Count > 0)
{
this.ParseError = true;
argList.ForEach(arg => error.Append("Unknown argument: '" + arg + "'.\r\n"));
}
}
catch (Exception ex)
{
this.ParseError = true;
error.Append("Error while trying to parse arguments.\r\n The error message is '" + ex.Message + "'.");
}
//save the collected errormessages
this.ParseErrorMessage = error.ToString();
}
}
///
/// The folder the user wants to delete, as a DirectoryInfo
///
/// The folder the user wants to delete, as a DirectoryInfo
public DirectoryInfo GetFolder()
{
DirectoryInfo folderDir = null;
//if no parameter was given or ".", then we use the current folder
if (string.IsNullOrEmpty(Folder) || Folder == ".")
{
folderDir = new FileInfo(Assembly.GetExecutingAssembly().Location).Directory;
}
else if (!Directory.Exists(Folder))
{
//if a folder parameter was given, but it doesn't exist, we throw an exception
throw new ArgumentException("The path '" + folderDir + "'is not a valid folder");
}
else
{
folderDir = new DirectoryInfo(this.Folder);
}
return folderDir;
}
}
}