Saving and loading data in a XNA windows game (XmlSerialization)
…with drag-and-drop code as a freebie
Here is a short introduction to saving and loading your gamedata in XNA using the built-in .net xml serialization. This only works for Windows games, not XBOX nor Windows Phone 7. But if you (like me) mostly code windows games, it’s very easy to use .
I’ve made a small helperclass “SerializationHelper” to assist with saving and loading data:
You use it by calling this code for saving objects:
SerializationHelper.Save(objectToSave);
and this code for loading them again:
MyObjectType theObject = SerializationHelper.Load<MyObjecttype>();
Sample of loading in a game:
SpaceShip _ship = SerializationHelper.Load<Spaceship>();
See it in action
Here you can see how I move objects in an XNA game and then exit the game. When I restart the game, the objects are where I left them when I last shut down.
I made a small class called GameThingy to show how to serialize/deserialize.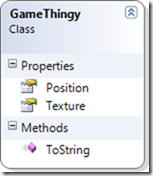
Classes for Xml serialization must have a default (parameterless) constructor. This means that you cannot use subclasses of GameComponent (including DrawableGameComponent) without a bit of code-trickery. So this is mainly usable for your own, homerolled classes.
Also be aware that some objects (Texture2D for example) are not serialized out, so you need to add this data yourself after you’ve deserialized the objects from xml:
//load the texture to use for the GameThingies Texture2D _xnafanTexture = Content.Load<Texture2D>("xnafan"); //load the list of GameThingy List<gamethingy> _gameThingies = SerializationHelper.Load<List<GameThingy>>(); //if there wasn't a list of _gameThingies (file didn't exist) if (_gameThingies == null) { //create a new, empty list _gameThingies = new List<GameThingy>(); } //set the Texture on all gamethingies _gameThingies.ForEach(t => t.Texture = _xnafanTexture);
Here's a more thorough walkthrough of the code:
January 30th, 2014 at 12:18
Hai sir, i followed your tutorial, but i getting an error while trying to save the data. What i was trying to save is saving the character, the tiles, and the items which these three from your tutorial.
The "simple platformer in xna" and "easily drag and drop"
Could you help me sir to fix this error?
Thank you very much!
January 30th, 2014 at 13:07
Hai sir, the error was solved, but right now i am unable to save the data, the xml file is there on "Debug" folder, but inside of the file, there is nothing, like stored information of the character position.. Why is it like that sir?
January 31st, 2014 at 10:54
Hi Frendy
I am going to have to see your code to figure out what is wrong.
I suggest you post here (http://xboxforums.create.msdn.com/forums/32.aspx) in the XNA forums, so I and other XNA developers can help you out.
Kind regards - Jakob
November 12th, 2014 at 20:25
Hi,
I have used your SerializationHelper class to save and load a List. This all works fine in VS2010 in both Debug and Release modes, but for some reason it crashes the published version. My game can't seem to save or load once published using VS. If I disable the loading it all works.
Any idea why this might be happening?
Thanks.
November 21st, 2014 at 09:28
Hi
Nope, it should work fine even though you've published it (I guess you're using Click-Once?).
What is the error message?
Perhaps the user running the program doesn't have the necessary permissions to save?
If you send me the published version I'll see if I can figure it out
Kind regards - Jakob
P.S. Sorry for the late answer, for some reason WordPress stopped notifying me of comments ... :-/